Introduction
This post will cover the basics of JSON, how to work with JSON in JavaScript, and practical examples. JSON (JavaScript Object Notation) is a lightweight data interchange format that’s easy for humans to read and write and easy for machines to parse and generate.
Working with JSON in JavaScript
1. What is JSON?
JSON is a text format for storing and transporting data. It’s often used when data is sent from a server to a web page. JSON data is written as key-value pairs, similar to JavaScript object literals:
//json
{
"name": "John",
"age": 30,
"isStudent": true
}
2. JSON Syntax
JSON syntax rules include:
- Data is in key-value pairs.
- Data is separated by commas.
- Curly braces hold objects.
- Square brackets hold arrays.
3. Parsing JSON
To convert a JSON string into a JavaScript object, use JSON.parse
:
//javascript
let jsonString = '{"name": "Alice", "age": 25, "isStudent": false}';
let jsonObj = JSON.parse(jsonString);
console.log(jsonObj); // Output: { name: 'Alice', age: 25, isStudent: false }
4. Converting Objects to JSON
To convert a JavaScript object into a JSON string, use JSON.stringify
:
//javascript
let obj = { name: 'Bob', age: 22, isStudent: true };
let jsonString = JSON.stringify(obj);
console.log(jsonString); // Output: '{"name":"Bob","age":22,"isStudent":true}'
5. Practical Examples
Let’s see some practical examples of working with JSON:
- Parsing JSON from an API Response:
//javascript
let response = '{"status": "success", "data": {"id": 1, "name": "John Doe"}}';
let parsedResponse = JSON.parse(response);
console.log(parsedResponse.data.name); // Output: John Doe
- Stringifying Data for API Requests:
//javascript
let data = {
title: 'JavaScript Basics',
author: 'Jane Smith',
published: true
};
let jsonString = JSON.stringify(data);
console.log(jsonString); // Output: '{"title":"JavaScript Basics","author":"Jane Smith","published":true}'
Conclusion
Understanding JSON is essential for working with data in JavaScript, especially when dealing with APIs. By mastering JSON parsing and stringifying, you can effectively handle data interchange in your web applications.
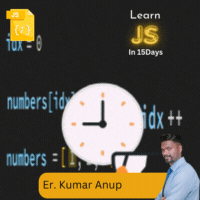
If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.