Introduction to Asynchronous JavaScript
Asynchronous JavaScript allows you to perform tasks without blocking the main thread, enabling smoother user experiences. This post will cover the basics of asynchronous programming, including callbacks, promises, and async/await.
1. Understanding Asynchronous Programming
Asynchronous programming allows tasks to run concurrently, improving the efficiency and responsiveness of your applications.
2. Callbacks
A callback is a function passed into another function as an argument, executed after the main function has finished:
//javascript
function fetchData(callback) {
setTimeout(function() {
callback('Data loaded');
}, 2000);
}
fetchData(function(message) {
console.log(message); // Output: Data loaded (after 2 seconds)
});
3. Promises
Promises provide a more powerful way to handle asynchronous operations, offering more control over success and failure cases:
//javascript
let promise = new Promise(function(resolve, reject) {
setTimeout(function() {
resolve('Promise resolved');
}, 2000);
});
promise.then(function(message) {
console.log(message); // Output: Promise resolved (after 2 seconds)
}).catch(function(error) {
console.error(error);
});
4. Async/Await
Async/await is a modern syntax for handling promises, making asynchronous code look more like synchronous code:
//javascript
async function fetchData() {
let promise = new Promise(function(resolve, reject) {
setTimeout(function() {
resolve('Data loaded');
}, 2000);
});
let result = await promise;
console.log(result); // Output: Data loaded (after 2 seconds)
}
fetchData();
5. Practical Examples
Let’s see some practical examples of asynchronous JavaScript:
- Fetching Data from an API:
//javascript
async function getData() {
try {
let response = await fetch('https://api.example.com/data');
let data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
getData();
- Simulating Asynchronous Operations:
//javascript
function simulateAsyncOperation() {
return new Promise(function(resolve) {
setTimeout(function() {
resolve('Operation complete');
}, 3000);
});
}
async function run() {
let result = await simulateAsyncOperation();
console.log(result); // Output: Operation complete (after 3 seconds)
}
run();
Conclusion
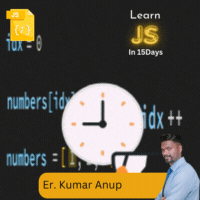
Asynchronous programming is crucial for building responsive web applications. By mastering callbacks, promises, and async/await, you can handle asynchronous operations effectively and improve the user experience.
If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.