Introduction
Control structures and loops in javaScript are fundamental programming concepts that allow you to control the flow of your code and repeat tasks. This post will cover conditional statements and various looping structures in JavaScript.
Today is day 11 and here we will learn Control Structures and Loops in JavaScript. Lets Start
1. Conditional Statements (if, else, switch)
Conditional statements allow you to perform different actions based on different conditions:
- if Statement:
//javascript
let age = 18;
if (age >= 18) {
console.log('You are an adult');
}
- if else Statement:
//javascript
let age = 16;
if (age >= 18) {
console.log('You are an adult');
} else {
console.log('You are a minor');
}
- if…else if…else Statement:
//javascript
let score = 85;
if (score >= 90) {
console.log('Grade: A');
} else if (score >= 80) {
console.log('Grade: B');
} else {
console.log('Grade: C');
}
- switch Statement:
//javascript
let day = 3;
switch (day) {
case 1:
console.log('Monday');
break;
case 2:
console.log('Tuesday');
break;
case 3:
console.log('Wednesday');
break;
default:
console.log('Invalid day');
}
2. Looping Structures (for, while, do-while)
Loops allow you to repeat a block of code multiple times:
- for Loop:
//javascript
for (let i = 0; i < 5; i++) {
console.log(`Iteration ${i}`);
}
- while Loop:
//javascript
let i = 0;
while (i < 5) {
console.log(`Iteration ${i}`);
i++;
}
- do-while Loop:
//javascript
let i = 0;
do {
console.log(`Iteration ${i}`);
i++;
} while (i < 5);
3. Break and Continue
Control the flow within loops using break
and continue
:
- break Statement:
//javascript
for (let i = 0; i < 10; i++) {
if (i === 5) {
break;
}
console.log(`Iteration ${i}`);
}
// Output: Iteration 0, Iteration 1, Iteration 2, Iteration 3, Iteration 4
- continue Statement:
//javascript
for (let i = 0; i < 10; i++) {
if (i === 5) {
continue;
}
console.log(`Iteration ${i}`);
}
// Output: Iteration 0, Iteration 1, Iteration 2, Iteration 3, Iteration 4, Iteration 6, Iteration 7, Iteration 8, Iteration 9
4. Practical Examples
Let’s see some practical examples of using control structures and loops:
- Checking Even or Odd Numbers:
//javascript
let number = 10;
if (number % 2 === 0) {
console.log(`${number} is even`);
} else {
console.log(`${number} is odd`);
}
- Finding the Sum of an Array:
//javascript
let numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
console.log(`Sum: ${sum}`); // Output: Sum: 15
Conclusion
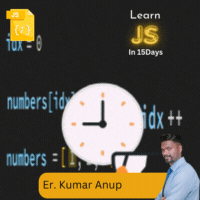
Control structures and loops are essential for writing flexible and efficient code. By mastering these concepts, you can control the flow of your programs and perform repetitive tasks effectively.
If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.