Building a Simple JavaScript Project
Building a project is a great way to apply what you’ve learned and solidify your understanding. In this post, we’ll create JavaScript Project a simple to-do list app using JavaScript.
1. Project Overview and Requirements
We’ll build a to-do list app that allows users to add, remove, and mark tasks as completed. Here’s what we’ll need:
- HTML for simple to-do list appthe structure
- CSS for styling
- JavaScript for functionality
2. Setting Up the Project Structure
Create the following files:
index.html
style.css
script.js
3. Creating and Manipulating the DOM
HTML (index.html
):
//html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>To-Do List App</title>
</head>
<body>
<div class="container">
<h1>To-Do List</h1>
<input type="text" id="taskInput" placeholder="Add a new task">
<button id="addTaskButton">Add Task</button>
<ul id="taskList"></ul>
</div>
<script src="script.js"></script>
</body>
</html>
CSS (style.css
):
//css
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
text-align: center;
}
input, button {
margin: 5px;
}
ul {
list-style: none;
padding: 0;
}
li {
background: #f4f4f4;
padding: 10px;
margin: 5px 0;
display: flex;
justify-content: space-between;
align-items: center;
}
.completed {
text-decoration: line-through;
color: grey;
}
JavaScript (script.js
):
//javascript
document.getElementById('addTaskButton').addEventListener('click', addTask);
function addTask() {
let taskInput = document.getElementById('taskInput');
let taskText = taskInput.value.trim();
if (taskText === '') return;
let taskList = document.getElementById('taskList');
let taskItem = document.createElement('li');
let taskContent = document.createElement('span');
taskContent.textContent = taskText;
taskItem.appendChild(taskContent);
let completeButton = document.createElement('button');
completeButton.textContent = 'Complete';
completeButton.addEventListener('click', function() {
taskContent.classList.toggle('completed');
});
taskItem.appendChild(completeButton);
let deleteButton = document.createElement('button');
deleteButton.textContent = 'Delete';
deleteButton.addEventListener('click', function() {
taskList.removeChild(taskItem);
});
taskItem.appendChild(deleteButton);
taskList.appendChild(taskItem);
taskInput.value = '';
}
4. Adding Interactivity with Event Handlers
We used event listeners to handle adding, completing, and deleting tasks. The addTask
function creates new list items with task text, complete, and delete buttons.
5. Final Touches and Deployment
Ensure your app looks good with CSS and functions correctly. You can deploy it using GitHub Pages or any static site hosting service.
Conclusion
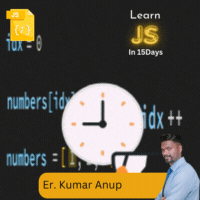
Building a to-do list app is a great way to apply your JavaScript skills in a practical project. It covers DOM manipulation, event handling, and basic styling, giving you a solid foundation to build more complex applications.
If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.