Introduction
Event handling is a crucial aspect of JavaScript, or say Handle events in javaScript allowing you to interact with users and respond to their actions. This post will cover how to handle events, add event listeners, and explore common event types.
so start writing blog to learn Handle events in javaScript
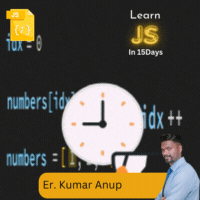
1. What are Events?
Events are actions or occurrences that happen in the browser, such as clicks, key presses, or mouse movements. JavaScript can be used to detect and respond to these events.
2. Adding Event Listeners
The addEventListener
method is used to attach an event handler to an element:
javascript Copy codedocument.getElementById('myButton').addEventListener('click', function() {
alert('Button clicked!');
});
3. Common Events
Some common events include:
- click: Triggered when an element is clicked.
//javascript
document.getElementById('myButton').addEventListener('click', function() {
console.log('Button clicked');
});
- mouseover: Triggered when the mouse hovers over an element.
//javascript
document.getElementById('myElement').addEventListener('mouseover', function() { console.log('Mouse over');
});
- keypress: Triggered when a key is pressed.
//javascript
document.addEventListener('keypress', function(event) {
console.log(`Key pressed: ${event.key}`);
});
4. Event Propagation (Bubbling and Capturing)
Event propagation determines the order in which events are handled in the DOM. There are two phases:
- Bubbling: The event is first captured and handled by the innermost element and then propagated to outer elements.
//javascript
document.getElementById('child').addEventListener('click', function() {
console.log('Child clicked');
});
document.getElementById('parent').addEventListener('click', function() {
console.log('Parent clicked');
});
// Clicking the child element will log 'Child clicked' followed by 'Parent clicked'
- Capturing: The event is first captured by the outermost element and propagated to the inner elements.
//javascript
document.getElementById('child').addEventListener('click', function() {
console.log('Child clicked');
}, true);
document.getElementById('parent').addEventListener('click', function() {
console.log('Parent clicked');
}, true);
// Clicking the child element will log 'Parent clicked' followed by 'Child clicked'
5. Practical Examples
Let’s see some practical examples of handling events:
- Changing Text on Click:
//javascript
document.getElementById('changeTextButton').addEventListener('click', function() {
document.getElementById('text').innerText = 'Text changed!';
});
- Hover Effect:
//javascript
document.getElementById('hoverElement').addEventListener('mouseover', function() {
this.style.backgroundColor = 'yellow';
});
document.getElementById('hoverElement').addEventListener('mouseout', function() {
this.style.backgroundColor = '';
});
Conclusion
Event handling allows you to create interactive web applications by responding to user actions. By mastering event listeners and understanding event propagation, you can build more dynamic and engaging user interfaces.

If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.