Start learning Error Handling and Debugging in JavaScript
Introduction
Error handling and debugging are critical skills for any JavaScript developer. This post will cover how to handle errors gracefully and use debugging tools to identify and fix issues in your code.
1. Common JavaScript Errors
Some common types of JavaScript errors include:
- Syntax Errors: Mistakes in the code syntax.
//javascript
console.log('Hello) // Missing closing quote
- Reference Errors: Attempting to access a variable that doesn’t exist.
//javascript
console.log(x); // x is not defined
- Type Errors: Performing an operation on a value of the wrong type.
//javascript
let num = 5;
num.toUpperCase(); // num.toUpperCase is not a function
2. Using try-catch for Error Handling
The try...catch
statement allows you to handle errors gracefully:
//javascript
try {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error('An error occurred:', error.message);
}
3. Debugging Tools and Techniques
Modern browsers provide powerful tools for debugging JavaScript code:
- Console Logging:
//javascript
console.log('Debug message');
- Browser DevTools:
- Use breakpoints to pause code execution and inspect variables.
- Step through code line by line to understand the flow.
- Debugger Statement:
//javascript
function test() {
debugger; // Execution will pause here
let x = 10;
console.log(x);
}
test();
4. Practical Examples
Let’s see some practical examples of error handling and debugging:
- Catching and Handling Errors:
//javascript
function divide(a, b) {
if (b === 0) {
throw new Error('Division by zero is not allowed');
}
return a / b;
}
try {
let result = divide(10, 0);
console.log(result);
} catch (error) {
console.error(error.message); // Output: Division by zero is not allowed
}
- Using Breakpoints in DevTools:
- Open the browser’s DevTools (usually by pressing F12).
- Go to the “Sources” tab.
- Set a breakpoint by clicking on the line number in your JavaScript file.
- Reload the page or trigger the JavaScript code.
- Inspect variables and the call stack when the breakpoint is hit.
Conclusion
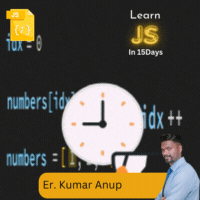
Effective error handling and debugging are essential for writing robust JavaScript applications. By mastering these techniques, you can identify and fix issues quickly, improving the reliability and performance of your code.
If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.