Introduction
The Dom in JavaScript (Document Object Model) is a programming interface for HTML and XML documents. It represents the page so that programs can change the document structure, style, and content. This post will cover the basics of the DOM and how to
So Start Reading DOM in JavaScript
1. What is the DOM?
The DOM is a hierarchical representation of a webpage. It allows JavaScript to access and manipulate elements on the page.
2. Selecting Elements
You can select elements in the DOM using various methods:
- getElementById: Selects an element by its ID.
//javascript
let element = document.getElementById('myElement');
- getElementsByClassName: Selects elements by their class name.
//javascript
let elements = document.getElementsByClassName('myClass');
- getElementsByTagName: Selects elements by their tag name.
//javascript
let elements = document.getElementsByTagName('p');
- querySelector: Selects the first element that matches a CSS selector.
//javascript
let element = document.querySelector('.myClass');
- querySelectorAll: Selects all elements that match a CSS selector.
//javascript
let elements = document.querySelectorAll('.myClass');
3. Modifying DOM Elements
You can modify the content and attributes of DOM elements:
- Changing Inner HTML:
//javascript
document.getElementById('myElement').innerHTML = '<p>New content</p>';
- Changing Attributes:
//javascript
document.getElementById('myLink').setAttribute('href', 'https://www.example.com');
- Changing Styles:
//javascript
document.getElementById('myElement').style.color = 'blue';
4. Traversing the DOM
You can navigate through the DOM tree using parent, child, and sibling properties:
- parentNode: Gets the parent node.
//javascript
let parent = document.getElementById('myElement').parentNode;
- childNodes: Gets a collection of child nodes.
//javascript
let children = document.getElementById('myElement').childNodes;
- nextSibling / previousSibling: Gets the next/previous sibling node.
//javascript
let nextSibling = document.getElementById('myElement').nextSibling;
5. Practical Examples
Let’s see some practical examples of manipulating the DOM:
- Adding a New Element:
//javascript
let newElement = document.createElement('div');
newElement.innerText = 'New Element';
document.body.appendChild(newElement);
- Removing an Element:
//javascript
let element = document.getElementById('myElement');
element.parentNode.removeChild(element);
Conclusion
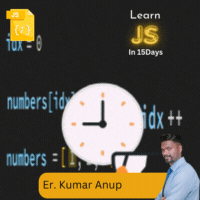
Understanding the DOM is crucial for creating dynamic and interactive web applications. By learning how to select, modify, and traverse DOM elements, you can manipulate web pages and enhance user experiences effectively.
If you haven’t read our last day blog on the basic introduction to JavaScript, click here to read our Blogs. want to connect me on linkdin.